注意
转到末尾下载完整的示例代码。或者通过 Binder 在您的浏览器中运行此示例
将灰度滤镜应用于 RGB 图像#
有许多滤波器被设计用于灰度图像,但不适用于彩色图像。为了简化创建可适应 RGB 图像的函数的过程,scikit-image 提供了 adapt_rgb
装饰器。
要真正使用 adapt_rgb
装饰器,您必须决定如何调整 RGB 图像以与灰度滤波器一起使用。 有两个预定义的处理程序
each_channel
将每个 RGB 通道逐个传递给滤波器,并将结果缝合回 RGB 图像。
hsv_value
将 RGB 图像转换为 HSV,并将值通道传递给滤波器。 过滤后的结果被插入回 HSV 图像并转换回 RGB。
下面,我们演示在几个灰度滤波器上使用 adapt_rgb
from skimage.color.adapt_rgb import adapt_rgb, each_channel, hsv_value
from skimage import filters
@adapt_rgb(each_channel)
def sobel_each(image):
return filters.sobel(image)
@adapt_rgb(hsv_value)
def sobel_hsv(image):
return filters.sobel(image)
我们可以像正常使用这些函数一样使用它们,但现在它们可以同时处理灰度图像和彩色图像。 让我们用彩色图像绘制结果
from skimage import data
from skimage.exposure import rescale_intensity
import matplotlib.pyplot as plt
image = data.astronaut()
fig, (ax_each, ax_hsv) = plt.subplots(ncols=2, figsize=(14, 7))
# We use 1 - sobel_each(image) but this won't work if image is not normalized
ax_each.imshow(rescale_intensity(1 - sobel_each(image)))
ax_each.set_xticks([]), ax_each.set_yticks([])
ax_each.set_title("Sobel filter computed\n on individual RGB channels")
# We use 1 - sobel_hsv(image) but this won't work if image is not normalized
ax_hsv.imshow(rescale_intensity(1 - sobel_hsv(image)))
ax_hsv.set_xticks([]), ax_hsv.set_yticks([])
ax_hsv.set_title("Sobel filter computed\n on (V)alue converted image (HSV)")
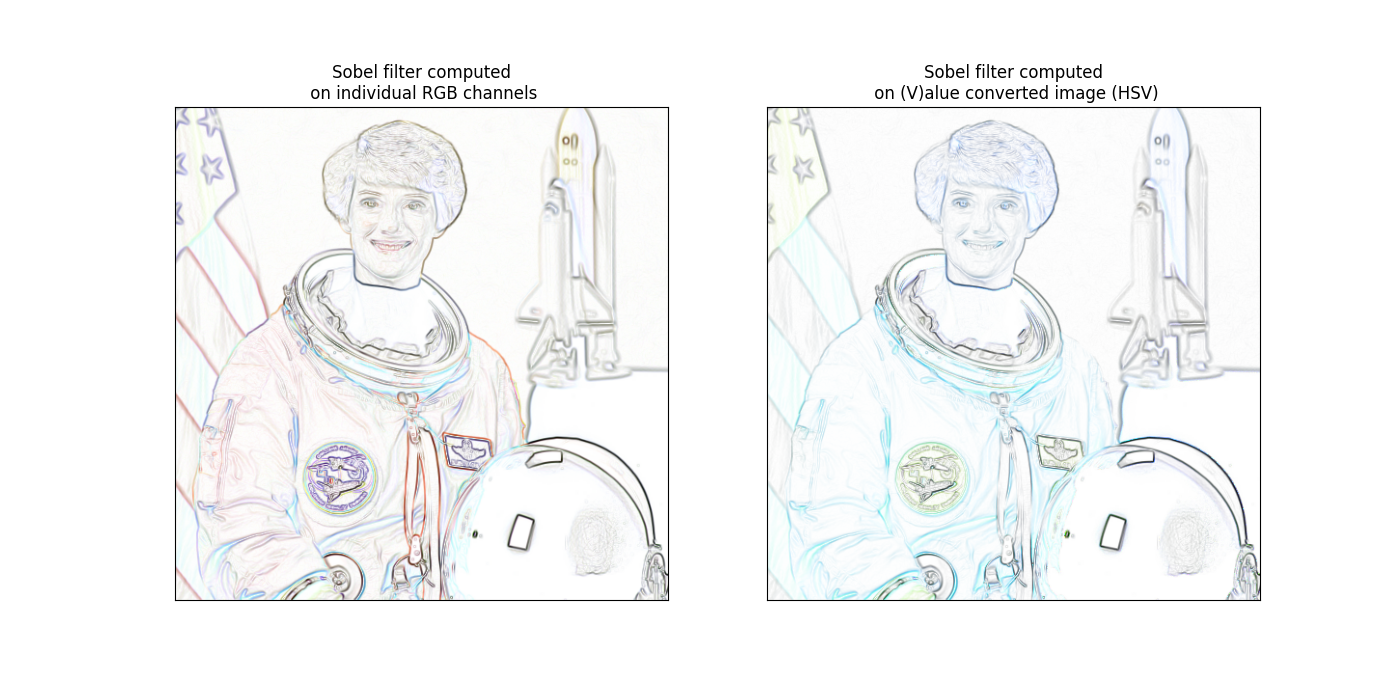
Text(0.5, 1.0, 'Sobel filter computed\n on (V)alue converted image (HSV)')
请注意,值滤波图像的结果保留了原始图像的颜色,但通道滤波图像以更令人惊讶的方式组合。 在其他常见情况下,例如平滑,通道滤波图像将产生比值滤波图像更好的结果。
您还可以为 adapt_rgb
创建自己的处理程序函数。 为此,只需创建一个具有以下签名的函数
请注意,adapt_rgb
处理程序是为图像是第一个参数的滤波器编写的。
作为一个非常简单的示例,我们可以将任何 RGB 图像转换为灰度,然后返回过滤后的结果
创建使用 *args
和 **kwargs
将参数传递给过滤器的签名非常重要,这样装饰后的函数可以有任意数量的位置和关键字参数。
最后,我们可以像以前一样将此处理程序与 adapt_rgb
一起使用
@adapt_rgb(as_gray)
def sobel_gray(image):
return filters.sobel(image)
fig, ax = plt.subplots(ncols=1, nrows=1, figsize=(7, 7))
# We use 1 - sobel_gray(image) but this won't work if image is not normalized
ax.imshow(rescale_intensity(1 - sobel_gray(image)), cmap=plt.cm.gray)
ax.set_xticks([]), ax.set_yticks([])
ax.set_title("Sobel filter computed\n on the converted grayscale image")
plt.show()
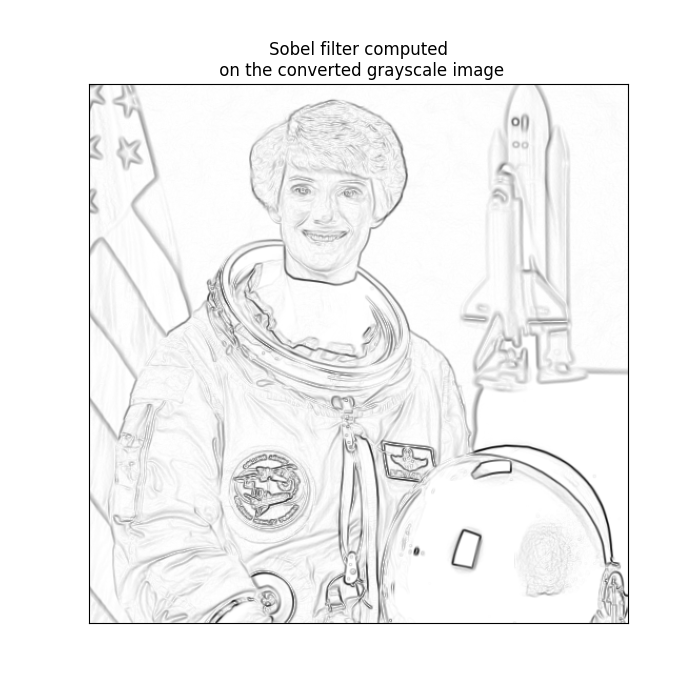
注意
一个非常简单的数组形状检查用于检测 RGB 图像,因此不建议将 adapt_rgb
用于支持 3D 体积或非 RGB 空间中的彩色图像的函数。
脚本的总运行时间:(0 分 2.019 秒)