注意
转到末尾下载完整的示例代码。或通过 Binder 在浏览器中运行此示例
直线霍夫变换#
霍夫变换最简单的形式是检测直线的方法 [1]。
在以下示例中,我们构造一个具有直线交点的图像。然后,我们使用霍夫变换。探索可能穿过图像的直线的参数空间。
算法概述#
通常,直线被参数化为 \(y = mx + c\),其中梯度为 \(m\),y 轴截距为 c
。然而,这意味着对于垂直线,\(m\) 会变为无穷大。因此,我们构造一个垂直于直线并通向原点的线段。直线由该线段的长度 \(r\) 以及它与 x 轴所成的角度 \(\theta\) 表示。
霍夫变换构造一个表示参数空间的直方图数组(即,对于半径的 \(M\) 个不同值和 \(\theta\) 的 \(N\) 个不同值,是一个 \(M \times N\) 矩阵)。对于每个参数组合 \(r\) 和 \(\theta\),我们然后找到输入图像中与相应直线接近的非零像素的数量,并在位置 \((r, \theta)\) 处适当地增加数组。
我们可以将每个非零像素视为“投票”给潜在的直线候选者。得到的直方图中的局部最大值表示最可能的直线的参数。在我们的示例中,最大值出现在 45 度和 135 度,对应于每条直线的法向量角度。
另一种方法是渐进概率霍夫变换 [2]。它基于以下假设:使用随机子集的投票点可以很好地近似实际结果,并且可以通过沿着连通分量行走在投票过程中提取直线。这返回每个线段的起点和终点,这很有用。
函数 probabilistic_hough
有三个参数:应用于霍夫累加器的通用阈值、最小线长和影响线合并的线间隙。在下面的示例中,我们找到长度大于 10 且间隙小于 3 像素的直线。
参考文献#
直线霍夫变换#
import numpy as np
from skimage.transform import hough_line, hough_line_peaks
from skimage.feature import canny
from skimage.draw import line as draw_line
from skimage import data
import matplotlib.pyplot as plt
from matplotlib import cm
# Constructing test image
image = np.zeros((200, 200))
idx = np.arange(25, 175)
image[idx, idx] = 255
image[draw_line(45, 25, 25, 175)] = 255
image[draw_line(25, 135, 175, 155)] = 255
# Classic straight-line Hough transform
# Set a precision of 0.5 degree.
tested_angles = np.linspace(-np.pi / 2, np.pi / 2, 360, endpoint=False)
h, theta, d = hough_line(image, theta=tested_angles)
# Generating figure 1
fig, axes = plt.subplots(1, 3, figsize=(15, 6))
ax = axes.ravel()
ax[0].imshow(image, cmap=cm.gray)
ax[0].set_title('Input image')
ax[0].set_axis_off()
angle_step = 0.5 * np.diff(theta).mean()
d_step = 0.5 * np.diff(d).mean()
bounds = [
np.rad2deg(theta[0] - angle_step),
np.rad2deg(theta[-1] + angle_step),
d[-1] + d_step,
d[0] - d_step,
]
ax[1].imshow(np.log(1 + h), extent=bounds, cmap=cm.gray, aspect=1 / 1.5)
ax[1].set_title('Hough transform')
ax[1].set_xlabel('Angles (degrees)')
ax[1].set_ylabel('Distance (pixels)')
ax[1].axis('image')
ax[2].imshow(image, cmap=cm.gray)
ax[2].set_ylim((image.shape[0], 0))
ax[2].set_axis_off()
ax[2].set_title('Detected lines')
for _, angle, dist in zip(*hough_line_peaks(h, theta, d)):
(x0, y0) = dist * np.array([np.cos(angle), np.sin(angle)])
ax[2].axline((x0, y0), slope=np.tan(angle + np.pi / 2))
plt.tight_layout()
plt.show()
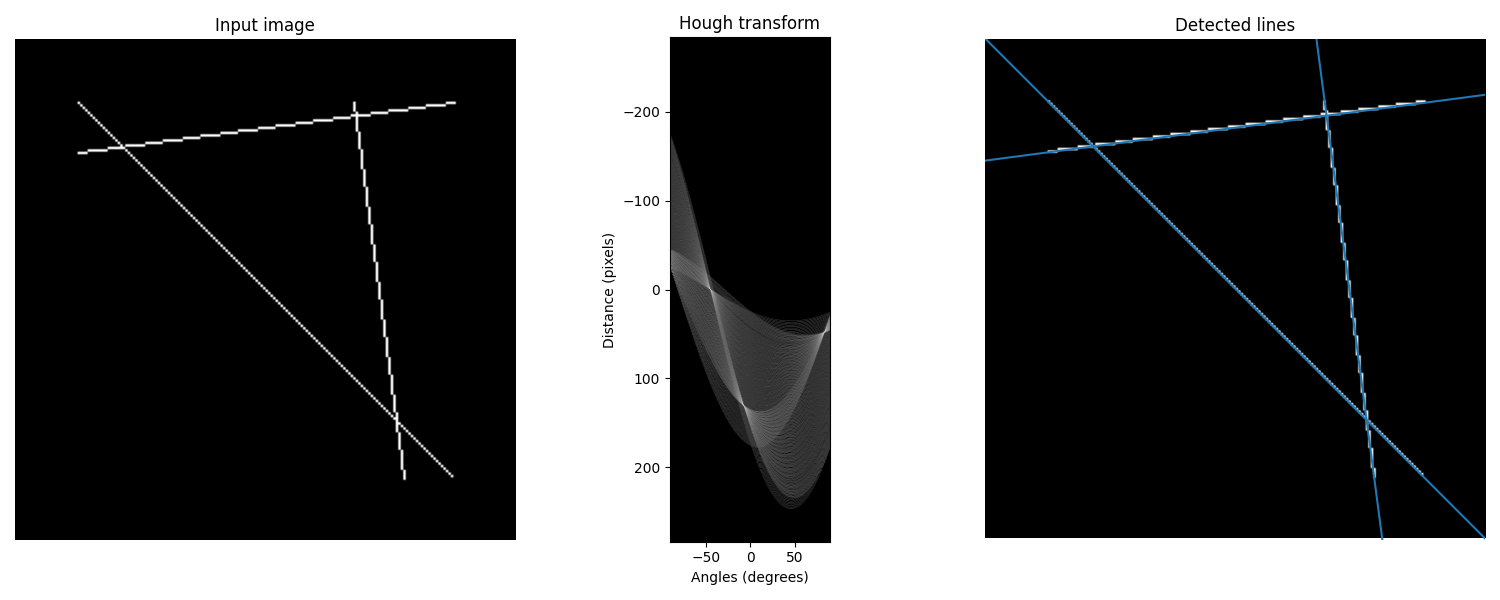
概率霍夫变换#
from skimage.transform import probabilistic_hough_line
# Line finding using the Probabilistic Hough Transform
image = data.camera()
edges = canny(image, 2, 1, 25)
lines = probabilistic_hough_line(edges, threshold=10, line_length=5, line_gap=3)
# Generating figure 2
fig, axes = plt.subplots(1, 3, figsize=(15, 5), sharex=True, sharey=True)
ax = axes.ravel()
ax[0].imshow(image, cmap=cm.gray)
ax[0].set_title('Input image')
ax[1].imshow(edges, cmap=cm.gray)
ax[1].set_title('Canny edges')
ax[2].imshow(edges * 0)
for line in lines:
p0, p1 = line
ax[2].plot((p0[0], p1[0]), (p0[1], p1[1]))
ax[2].set_xlim((0, image.shape[1]))
ax[2].set_ylim((image.shape[0], 0))
ax[2].set_title('Probabilistic Hough')
for a in ax:
a.set_axis_off()
plt.tight_layout()
plt.show()
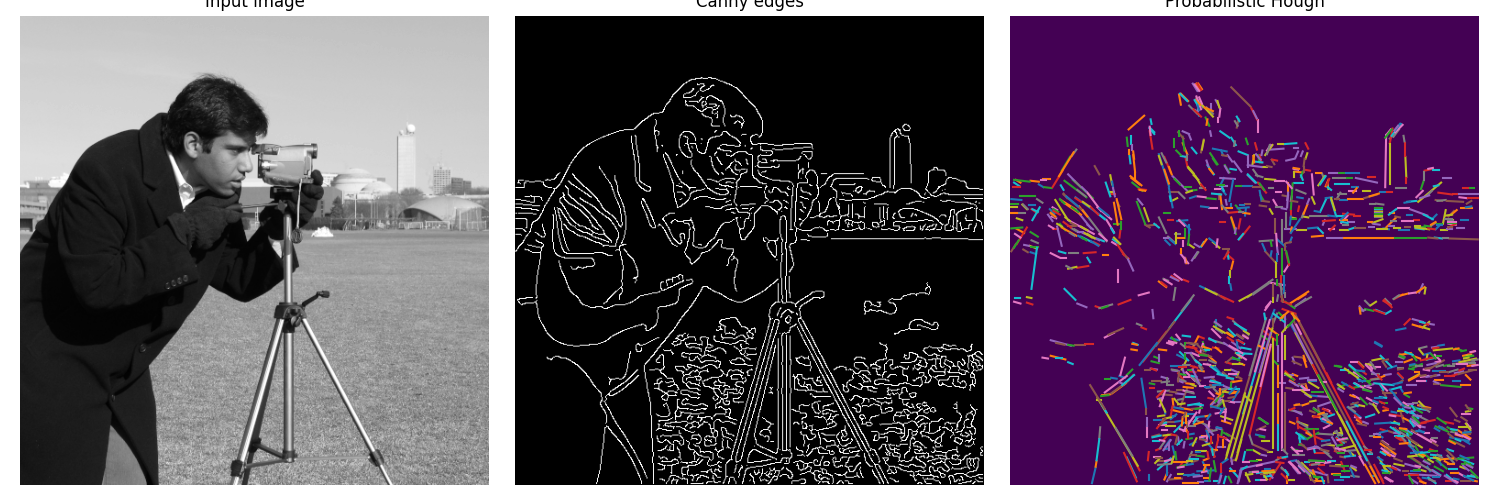
脚本的总运行时间:(0 分钟 3.383 秒)