注意
转到末尾下载完整的示例代码。或通过 Binder 在您的浏览器中运行此示例
掩模归一化互相关#
在此示例中,我们使用掩模归一化互相关来识别两个包含无效数据的相似图像之间的相对位移。
在这种情况下,不能简单地在计算互相关之前掩模图像,因为掩模会影响计算。必须从互相关中删除掩模的影响,如 [1] 中所述。
在此示例中,我们注册两个图像之间的平移。但是,其中一个图像约有 25% 的像素已损坏。
import numpy as np
import matplotlib.pyplot as plt
from skimage import data, draw
from skimage.registration import phase_cross_correlation
from scipy import ndimage as ndi
定义图像中无效的区域。无效像素的概率为 25%。这可能是由于检测器故障,或不受平移影响的边缘(例如,窗口中的移动对象)。有关更多示例,请参阅参考论文
image = data.camera()
shift = (-22, 13)
rng = np.random.default_rng()
corrupted_pixels = rng.choice([False, True], size=image.shape, p=[0.25, 0.75])
# The shift corresponds to the pixel offset relative to the reference image
offset_image = ndi.shift(image, shift)
offset_image *= corrupted_pixels
print(f'Known offset (row, col): {shift}')
# Determine what the mask is based on which pixels are invalid
# In this case, we know what the mask should be since we corrupted
# the pixels ourselves
mask = corrupted_pixels
detected_shift, _, _ = phase_cross_correlation(image, offset_image, reference_mask=mask)
print(f'Detected pixel offset (row, col): {-detected_shift}')
fig, (ax1, ax2, ax3) = plt.subplots(1, 3, sharex=True, sharey=True, figsize=(8, 3))
ax1.imshow(image, cmap='gray')
ax1.set_axis_off()
ax1.set_title('Reference image')
ax2.imshow(offset_image.real, cmap='gray')
ax2.set_axis_off()
ax2.set_title('Corrupted, offset image')
ax3.imshow(mask, cmap='gray')
ax3.set_axis_off()
ax3.set_title('Masked pixels')
plt.show()
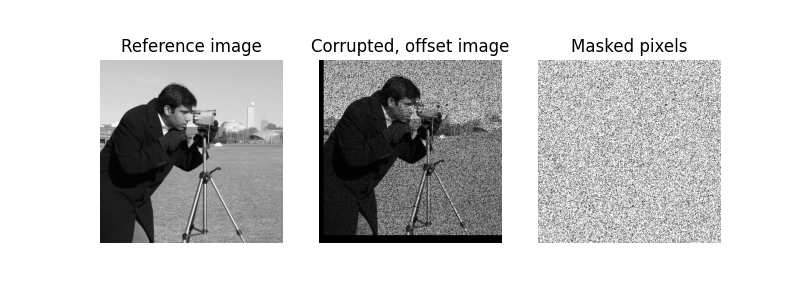
Known offset (row, col): (-22, 13)
Detected pixel offset (row, col): [-22. 13.]
实心掩模是另一个说明示例。在这种情况下,我们有一个图像的有限视图和一个偏移图像。这些图像的掩模不必相同。phase_cross_correlation
函数将正确识别应比较图像的哪一部分。
image = data.camera()
shift = (-22, 13)
rr1, cc1 = draw.ellipse(259, 248, r_radius=125, c_radius=100, shape=image.shape)
rr2, cc2 = draw.ellipse(300, 200, r_radius=110, c_radius=180, shape=image.shape)
mask1 = np.zeros_like(image, dtype=bool)
mask2 = np.zeros_like(image, dtype=bool)
mask1[rr1, cc1] = True
mask2[rr2, cc2] = True
offset_image = ndi.shift(image, shift)
image *= mask1
offset_image *= mask2
print(f'Known offset (row, col): {shift}')
detected_shift, _, _ = phase_cross_correlation(
image, offset_image, reference_mask=mask1, moving_mask=mask2
)
print(f'Detected pixel offset (row, col): {-detected_shift}')
fig = plt.figure(figsize=(8, 3))
ax1 = plt.subplot(1, 2, 1)
ax2 = plt.subplot(1, 2, 2, sharex=ax1, sharey=ax1)
ax1.imshow(image, cmap='gray')
ax1.set_axis_off()
ax1.set_title('Reference image')
ax2.imshow(offset_image.real, cmap='gray')
ax2.set_axis_off()
ax2.set_title('Masked, offset image')
plt.show()
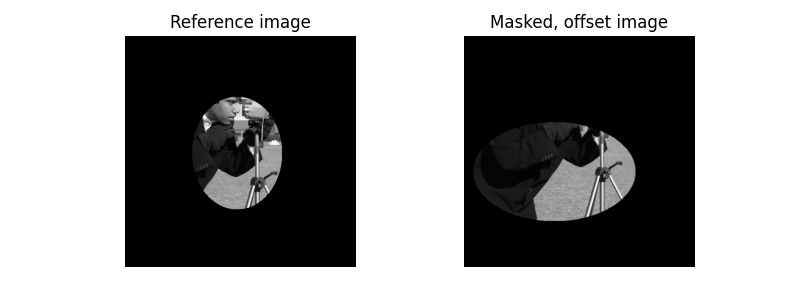
Known offset (row, col): (-22, 13)
Detected pixel offset (row, col): [-22. 13.]
脚本的总运行时间: (0 分钟 1.105 秒)